C++ for_each
Date: 2018-10-13Last modified: 2022-12-17
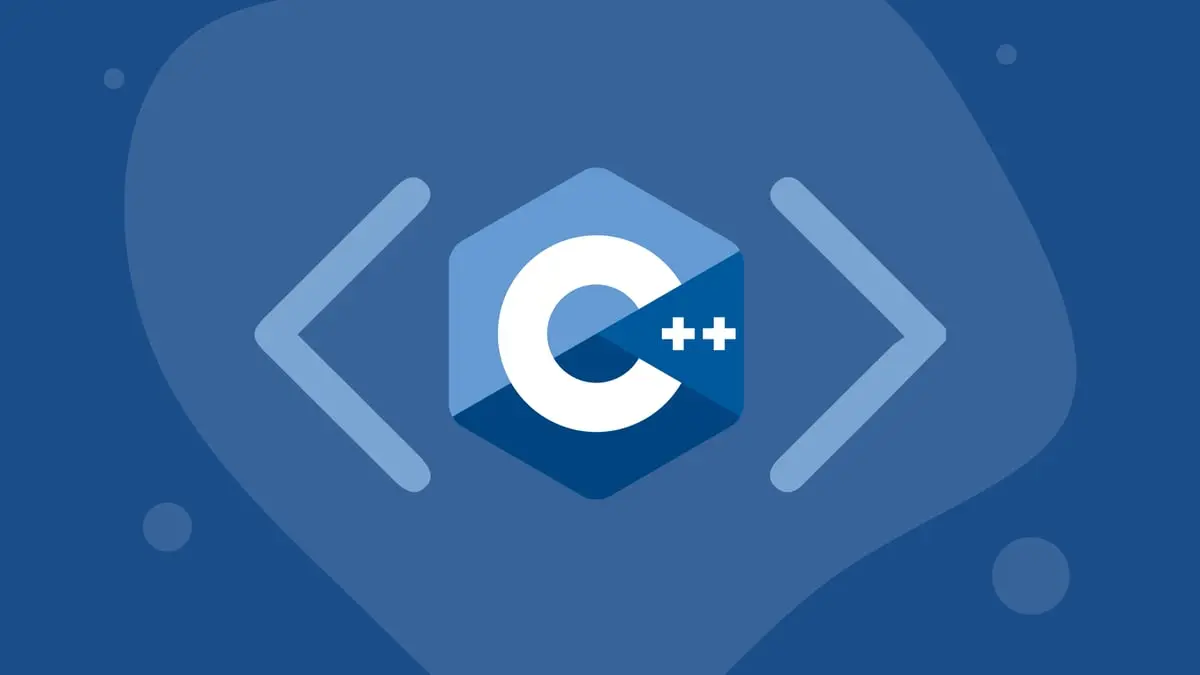
Table of contents
Lambda vs Object Function
The lambda function [](const int &e){}
is equivalent to an object
function like this:
struct FunctionObjectPrinter {
void operator()( const int &e )
{
cout << e << ", ";
}
};
The lambda function [prefix](const int &e){}
is equivalent to an object
function like this:
struct FunctionObjectPrinterWithPrefix {
explicit FunctionObjectPrinterWithPrefix( const string &prefix ) : mPrefix( prefix )
{
}
void operator()( const int &e )
{
cout << mPrefix << " " << e << ", ";
}
private:
string mPrefix;
};
👇
Note
for_each
does not use the function return value
list<int> v{ 1, 2, 3, 4, 5 };
Range operator
for( auto &e : v ) {
cout << e << ", ";
}
cout << "range operator" << endl;
Function object
FunctionObjectPrinter p;
for_each( begin( v ), end( v ), p );
cout << "function operator" << endl;
Inline lambda
for_each( begin( v ), end( v ), []( const int &e ) { cout << e << ", "; } );
cout << "inline lambda" << endl;
Lambda
auto print = []( const int &n ) { cout << n << ", "; };
for_each( begin( v ), end( v ), print );
cout << "lambda" << endl;
Range operator + lambda
for( auto &e : v ) {
print( e );
}
cout << "range operator + lambda" << endl;
## Output
```txt
1, 2, 3, 4, 5, range operator
1, 2, 3, 4, 5, function operator
1, 2, 3, 4, 5, inline lambda
1, 2, 3, 4, 5, lambda
1, 2, 3, 4, 5, range operator + lambda