C++ algorithm max_element and min_element
Date: 2022-12-26Last modified: 2022-12-30
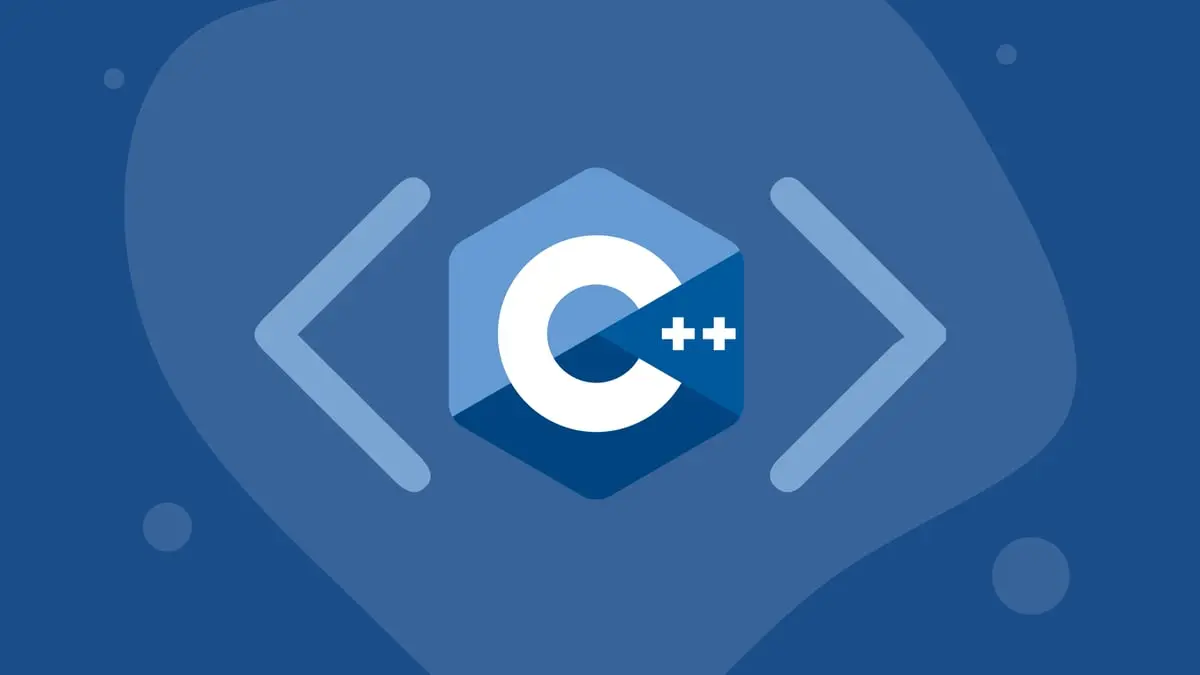
Table of contents
vector<int> vec = {18, 21, 42, 17, 0, -1, 21, 10, 10};
auto mx = max_element(begin(vec), end(vec)); // O(N)
auto mn = min_element(begin(vec), end(vec)); // O(N)
cout << "Max element is " << *mx << endl;
cout << "Min element is " << *mn << endl;
Print lambda:
auto print = [](const auto &vec, const string &msg) {
for (const auto &e : vec) {
cout << e << ' ';
}
cout << msg << endl;
};
The std::swap()
is a built-in function in C++ STL which swaps
the value of any two variables passed to it as parameters.
print(vec, "original vector");
std::swap(mn, mx); // swap iterator value, but not its position
cout << "Max element is " << *mx << endl;
cout << "Min element is " << *mn << endl;
print(vec, "after swap");
The std::iter_swap
swaps the values of the elements the given
iterators are pointing to.
std::iter_swap(mn, mx);
print(vec, "after iter_swap");
Possible output
Max element is 42
Min element is -1
18 21 42 17 0 -1 21 10 10 original vector
Max element is -1
Min element is 42
18 21 42 17 0 -1 21 10 10 after swap
18 21 -1 17 0 42 21 10 10 after iter_swap