C++ array
Date: 2020-01-04Last modified: 2022-12-27
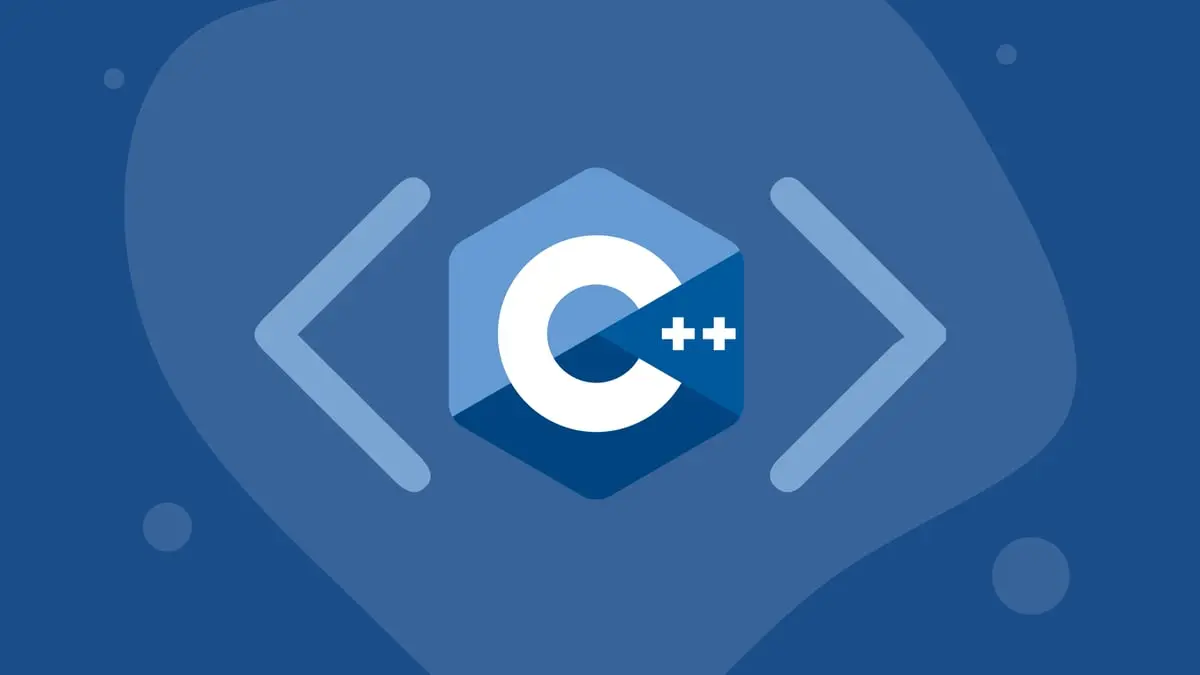
Exemplos de uso de std::array
Características
- é um wrapper para o array do C
- array oferece uma sintaxe mais amigável comparada com a array do C
- pode ser passado e retornado por valor
- pode ser passado e retornado por referência
- uso mais conveniente de
size
- uso mais conveniente de iteradores STL
- possui exatamente a mesma performace da array do C
Array vs Vector
array
é estático e possui tamanho definido em tempo de compilaçãovector
é dinâmico e é alocado noheap
Exemplos
#include <algorithm>
#include <array>
#include <iostream>
using namespace std;
// Multidimensionais
template <class T, size_t ROW, size_t COL>
using Matrix = std::array<std::array<T, COL>, ROW>;
template <class T, size_t I, size_t... J>
struct MultiDimArray {
using Nested = typename MultiDimArray<T, J...>::type;
using type = std::array<Nested, I>;
};
template <class T, size_t I>
struct MultiDimArray<T, I> {
using type = std::array<T, I>;
};
int main( int argc, char **argv )
{
auto print = []( const auto &arr ) {
cout << "{ ";
for( const auto &x : arr ) {
cout << x << " ";
}
cout << "}" << endl;
};
array<int, 3> a = {2, 1, 3};
int c[3] = {2, 1, 3}; // old C style
sort( a.begin(), a.end() );
print( a ); // { 1, 2, 3 }
sort( begin( c ), end( c ) ); // c == { 1, 2, 3 }
print( c );
for( auto &x : a ) {
x *= 2;
}
print( a ); // { 2, 4, 6 }
for( auto &x : c ) {
x *= 2;
}
print( c ); // { 2, 4, 6 }
array<array<int, 3>, 3> arr = {{{5, 8, 2}, {8, 3, 1}, {5, 3, 9}}};
cout << arr[2][2] << endl; // 9
Matrix<float, 3, 4> mat;
mat[1][2] = 5;
MultiDimArray<int, 2, 3, 4, 5>::type multi;
multi[1][1][1][1] = 5;
return 0;
}