C++ big endian vs little endian
Date: 2022-12-29Last modified: 2022-12-29
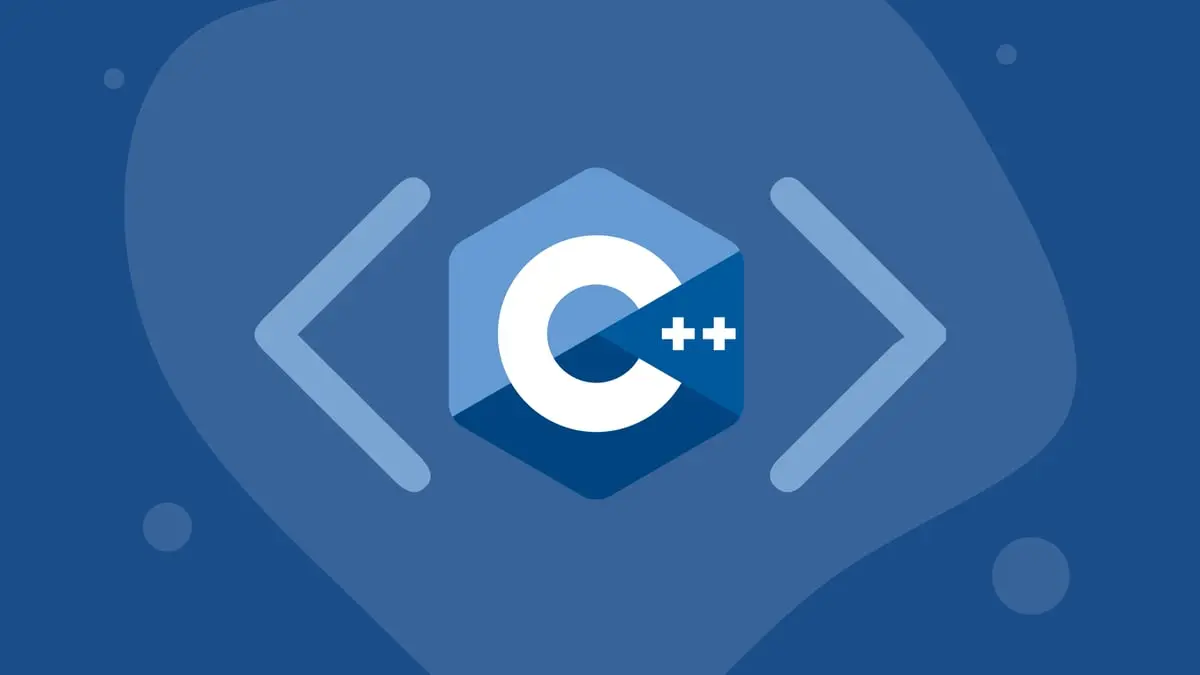
Table of contents
Byte significant
- LSB - Less significant byte
- Any change to will cause a small change in this number
- MSB - Most significant byte
- Any change to will cause a huge change in this number
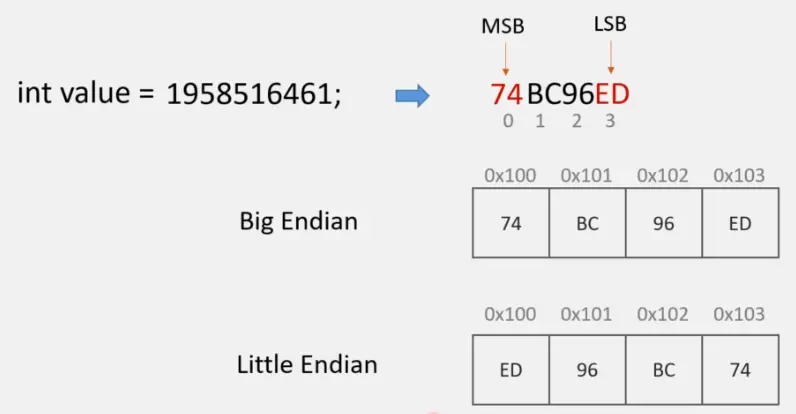
// uint32_t value = 1958516461;
uint32_t value = 0x01234567;
cout << fmt::format("Value printed in hex: {:08x}", value);
Any object in C++ can be reinterpreted as an array of bytes. If you
want to actually make a copy of the bytes into a separate array,
you can use std::copy
:
unsigned char bytes[sizeof value];
std::copy(
static_cast<const unsigned char *>(static_cast<const void *>(&value)),
static_cast<const unsigned char *>(static_cast<const void *>(&value)) +
sizeof value,
bytes);
// Print
cout << "\nBytes in memory (1): ";
for (const auto &byte : bytes) {
cout << fmt::format("{:02x} ", byte);
}
// Print
cout << "\nBytes in memory (2): " << hex;
copy(begin(bytes), end(bytes), std::ostream_iterator<int>(cout, " "));
When above program is run on little endian machine, gives “67
//-- When above program is run on little endian machine, gives “67
45 23 01” as output, while if it is run on big endian machine,
//-- 45 23 01” as output, while if it is run on big endian machine,
gives “01 23 45 67” as output.
//-- gives “01 23 45 67” as output.
How to detect endianness in C
{
unsigned int i = 1;
// i: 01 00 00 00 on little endian machines
// i: 00 00 00 01 on big endian machines
// c: ^^
char *c = (char *)&i;
if (*c) {
cout << "\nlittle-endian";
} else {
cout << "\nbig-endian";
}
// This code is contributed by rathbhupendra
}
How to detect endianness in C++
// #include <bit>
if constexpr (std::endian::native == std::endian::big) {
std::cout << "\nbig-endian";
} else if constexpr (std::endian::native == std::endian::little) {
std::cout << "\nlittle-endian";
} else {
std::cout << "\nmixed-endian";
}
Possible output
Value printed in hex: 01234567
Bytes in memory (1): 67 45 23 01
Bytes in memory (2): 67 45 23 1
little-endian
little-endian