C++ constructor_01
Date: 2020-02-25Last modified: 2025-01-12
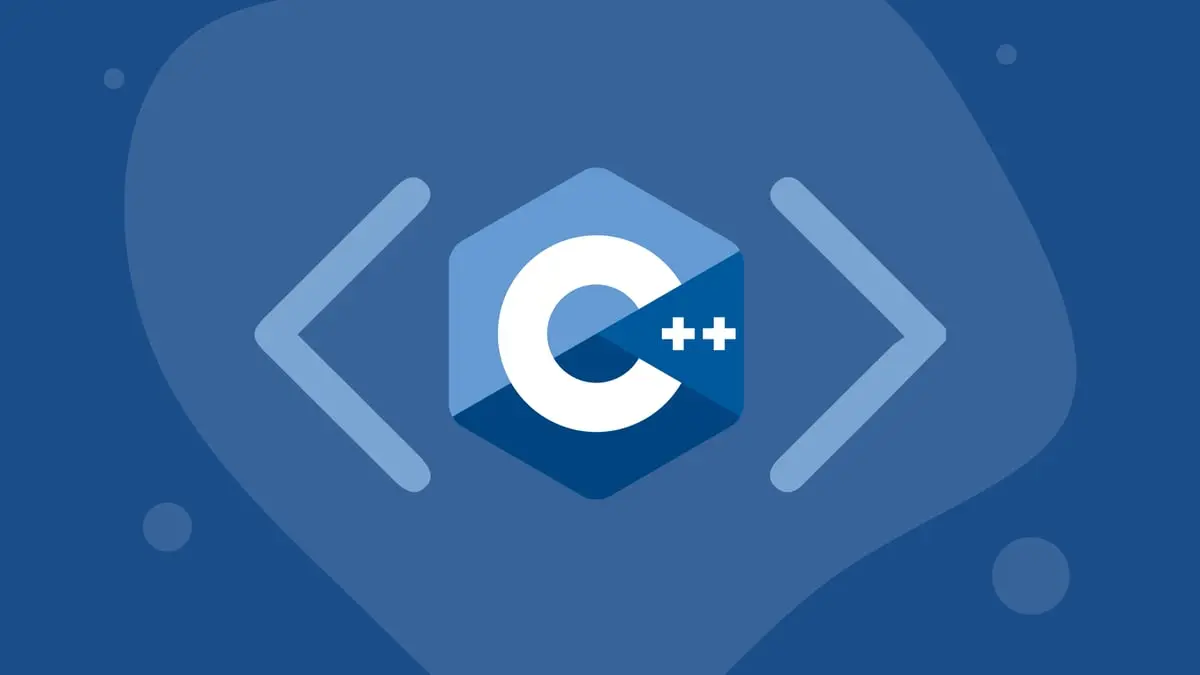
Table of contents
class A {
public:
A()
{
}
int i; // not initialized
int j = 5;
};
class B {
public:
B() = default;
int i; // will be initialized with 0
int j; // will be initialized with 0
};
int main( [[maybe_unused]] int argc, [[maybe_unused]] char **argv )
{
for( int i = 0; i < 5; ++i ) {
A *pa = new A();
B *pb = new B();
cout << "A.i = " << pa->i << " A.j = " << pa->j << " without default constructor" << endl;
cout << "B.i = " << pb->i << " B.j = " << pb->j << " with default constructor" << endl;
delete pa;
delete pb;
}
return 0;
}
Possible output
A.i = 0 A.j = 5 without default constructor
B.i = 0 B.j = 0 with default constructor
A.i = -161976930 A.j = 5 without default constructor
B.i = 0 B.j = 0 with default constructor
A.i = -161976834 A.j = 5 without default constructor
B.i = 0 B.j = 0 with default constructor
A.i = -161976930 A.j = 5 without default constructor
B.i = 0 B.j = 0 with default constructor
A.i = -161976834 A.j = 5 without default constructor
B.i = 0 B.j = 0 with default constructor