C++ convert operator
Date: 2023-01-02Last modified: 2023-01-03
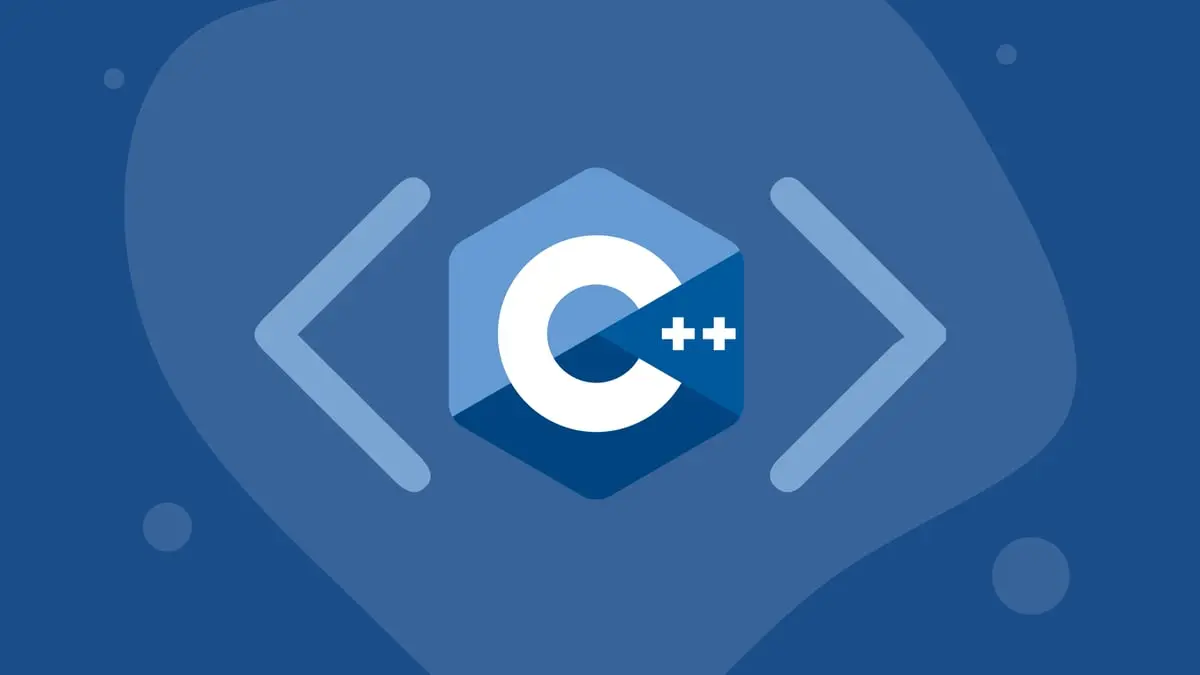
Table of contents
Converting to Type
works by overloading the Type()
operator.
class MyClass {
public:
operator Type() const {
// return something of type Type
}
};
For example, we could define a conversion of the MyVector
to a bool
to be false
if the vector is empty and true
otherwise.
MyVector v;
if (!v) { // call bool()
fmt::print("MyVector is empty\n");
}
v.add(42);
if (v) { // call bool()
fmt::print("First value on MyVector is {}\n", v[0]);
}
v.add(21);
v.add(7);
v.add(3);
string s = v;
fmt::print("MyVector converted to string is '{}'\n", s);
Possible output
bool() returns false
MyVector is empty
bool() returns true
First value on MyVector is 42
MyVector converted to string is '42, 21, 7, 3'