C++ declspec_01
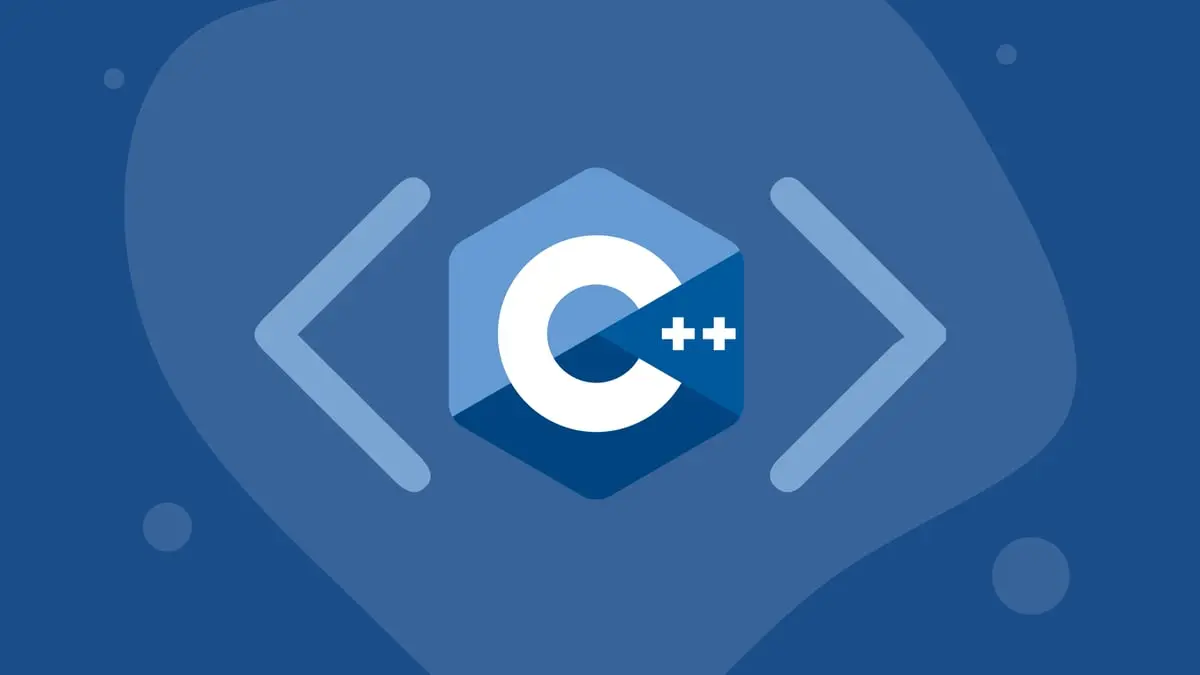
Table of contents
__declspec
is a C++ language extension that allows programmers
to specify various attributes or properties of functions and data
types in a Microsoft Windows environment. This extension is used
to indicate how a particular function or variable should be treated
by the compiler or the operating system.
The __declspec
extension provides a mechanism to specify various
attributes of C++ entities that are specific to the Windows operating
system, such as the calling convention, the export or import of
functions and data, and the memory allocation model.
Understanding how to use __declspec
can be beneficial for
developers who need to write code that interacts with the Windows
API, creates DLLs or shared libraries, or uses advanced memory
management techniques. In this introduction, we will explore
the different ways in which __declspec
can be used in C++
programming to optimize the performance and functionality of software
applications running on the Windows platform.
Não é uma extensão padrão e é válido somente para clang / msvc / intel.
Use as opções -fdeclspec
ou -fms-extensions
para habilitar
Get and Set
For example, consider a class with private data members that need
to be accessed through getter and setter functions. To ensure that
these functions are accessed efficiently, you can use __declspec
with the appropriate attribute.
class Person {
private:
int mAge{42};
public:
int getAge() const {
cout << "Person::getAge() called here" << endl;
return mAge;
}
void setAge(int value) {
cout << "Person::setAge(" << value << ") called here" << endl;
mAge = value;
}
__declspec(property(get = getAge, put = setAge)) int age;
};
Person person;
cout << person.age << endl; // Call person.getAge();
person.age = 20; // Call person.setAge(20);
Possible output
Person::getAge() called here
42
Person::setAge(20) called here