Forward List in C++
Date: 2023-01-30Last modified: 2023-02-01
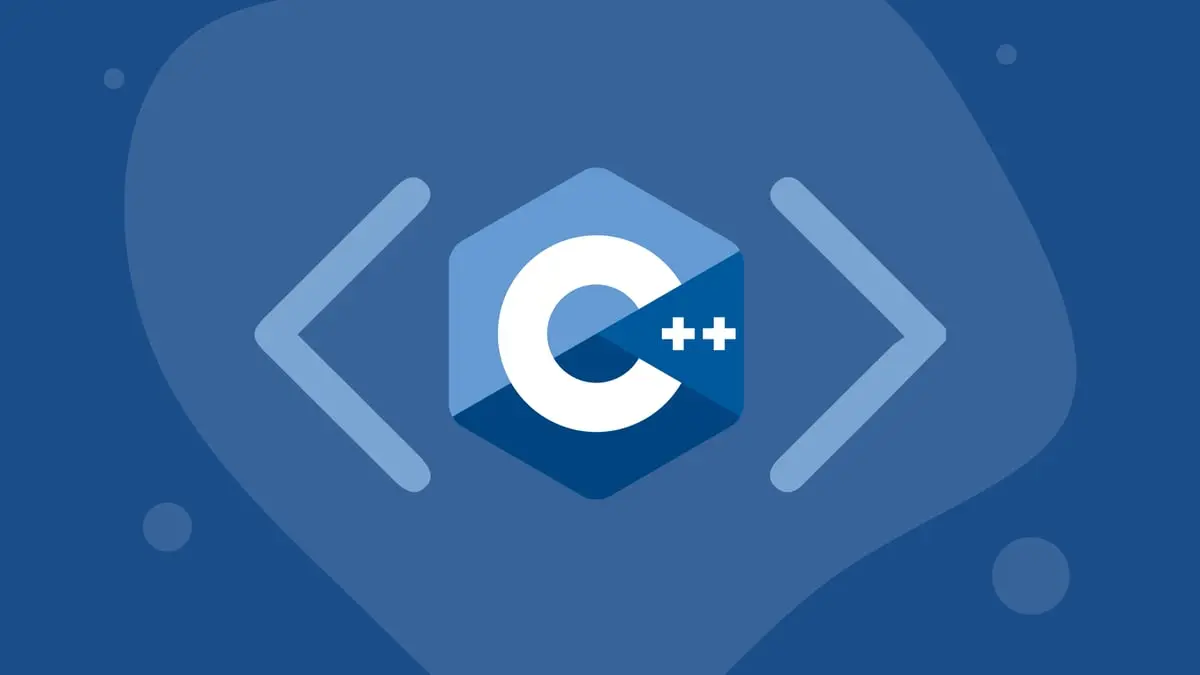
Table of contents
Introduction
- introduced from C+±11
- singly linked list
- can save space compared with
std::list
- cannot be iterated backwards
- don’t have
push_back
,pop_back
,emplace_back
,size
functions
forward_list<int> flist1;
forward_list<int> flist2;
forward_list<int> flist3;
flist1.assign({1, 2, 3, 4}); // 1, 2, 3, 4
flist2.assign(5, 10); // (size, default value) 10, 10, 10, 10, 10
flist3.assign(flist1.begin(), flist1.end()); // 1, 2, 3, 4
flist2.push_front(50); // 50, 10, 10, 10, 10, 10
flist2.emplace_front(60); // 60, 50, 10, 10, 10, 10, 10
flist3.pop_front(); // 2, 3, 4
flist3.insert_after(++flist3.begin(),
{200, 300, 400}); // 2, 3, 200, 300, 400, 4
flist3.erase_after(flist3.begin(),
next(flist3.begin(), 3)); // 2, 300, 400, 4
flist2.remove(10); // 60, 50
flist2.remove_if([](const auto &i) { return i > 55; }); // 50
cout << '\n';
for (const auto &i : flist1) {
cout << i << " ";
}
cout << '\n';
for (const auto &i : flist2) {
cout << i << " ";
}
cout << '\n';
for (const auto &i : flist3) {
cout << i << " ";
}
Possible output
1 2 3 4
50
2 300 400 4
References
- [Forward List in C++ | Set 1 (Introduction and Important