C++ hash
Date: 2022-12-27Last modified: 2022-12-27
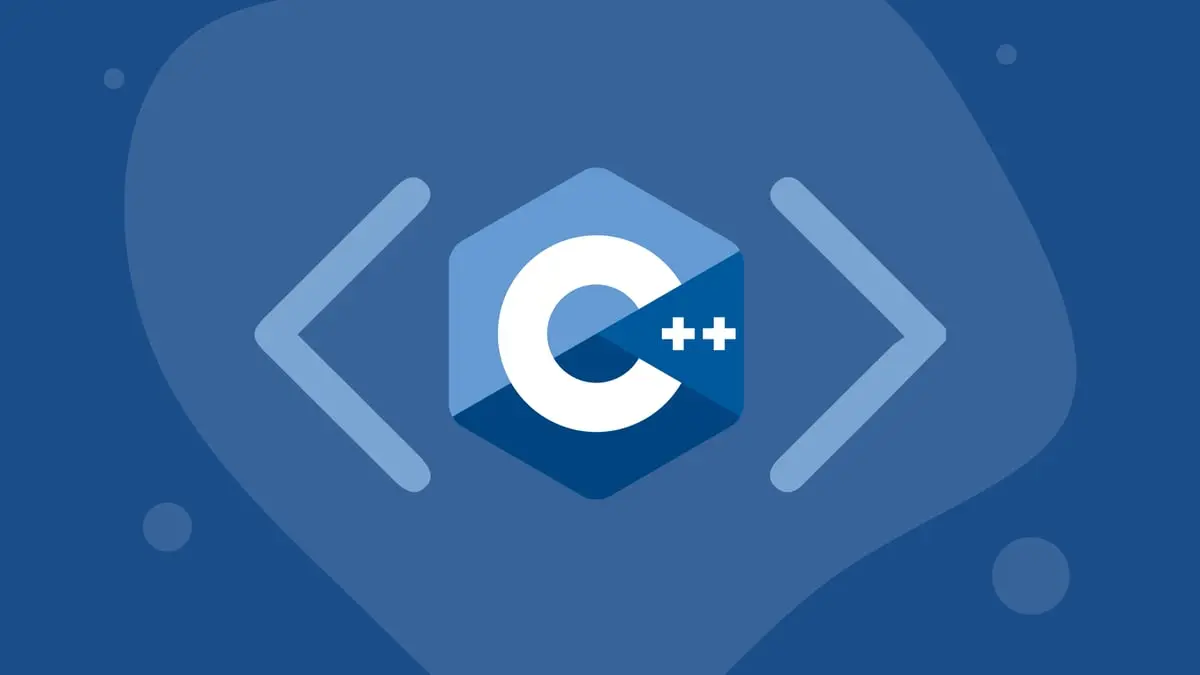
Table of contents
A basic struct for demonstration purpose:
struct S {
std::string first_name;
std::string last_name;
};
bool operator==(const S& lhs, const S& rhs) {
return lhs.first_name == rhs.first_name && lhs.last_name == rhs.last_name;
}
⚡
Tip
Custom hash can be a standalone function object.
struct MyHash {
std::size_t operator()(S const& s) const noexcept {
std::size_t h1 = std::hash<std::string>{}(s.first_name);
std::size_t h2 = std::hash<std::string>{}(s.last_name);
return h1 ^ (h2 << 1); // combine hashes
}
};
⚡
Tip
Custom specialization of std::hash can be injected in namespace std.
template <>
struct std::hash<S> {
std::size_t operator()(S const& s) const noexcept {
std::size_t h1 = std::hash<std::string>{}(s.first_name);
std::size_t h2 = std::hash<std::string>{}(s.last_name);
return h1 ^ (h2 << 1); // combine hashes
}
};
// size_t will take 32bits on 32bits systems
// size_t will take 64bits on 64bits systems
size_t h1 = hash<string>{}("Geraldo");
size_t h2 = hash<string>{}("geraldo");
size_t h3 = h1 ^ (h2 << 1);
size_t h4 = hash<char>{}('\n');
size_t h5 = hash<int>{}(10);
size_t h6 = hash<long>{}(10);
S obj = {"Geraldo", "Ribeiro"};
size_t h7 = hash<S>{}(obj);
cout << "h1 = " << h1 << '\n';
cout << "h2 = " << h2 << '\n';
cout << "h3 = " << h3 << '\n';
cout << "h4 = " << h4 << '\n';
cout << "h5 = " << h5 << '\n';
cout << "h6 = " << h6 << '\n';
cout << "h7 = " << h7 << '\n';
Possible output
h1 = 16517155802914804667
h2 = 15968437481030298971
h3 = 6777401911469705485
h4 = 10
h5 = 10
h6 = 10
h7 = 7993227047372965953