C++ GDB pretty print
Date: 2023-02-25Last modified: 2024-06-09
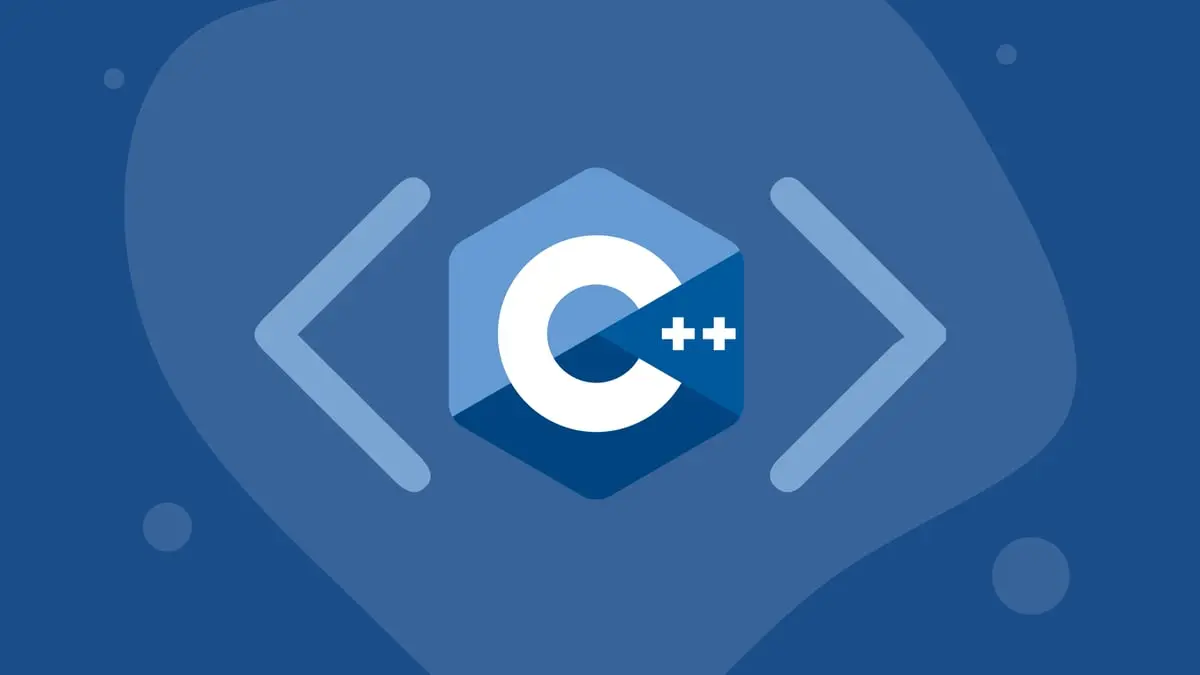
Table of contents
Pretty-Printer Introduction
Here is how a C++ std::string
looks without a pretty-printer:
(gdb) print s
$1 = {
static npos = 4294967295,
_M_dataplus = {
<std::allocator<char>> = {
<__gnu_cxx::new_allocator<char>> = {
<No data fields>}, <No data fields>
},
members of std::basic_string<char, std::char_traits<char>,
std::allocator<char> >::_Alloc_hider:
_M_p = 0x804a014 "abcd"
}
}
With a pretty-printer for std::string
only the contents are printed:
(gdb) print s
$2 = "abcd"
#include <stdlib.h>
typedef struct {
const char *first_name;
const char *last_name;
struct {
int day;
int month;
int year;
} dob;
char *comments;
} student;
student students[]
= { [0] = { .first_name = "Fred", .last_name = "Smith", .dob = { 1, 1, 1970 } },
[1]
= { .first_name = "Sarah",
.last_name = "G0T0",
.dob = { 1, 1, 2002 },
.comments = "Lorem ipsum enchanta constrata" } };
int main( void )
{
students[1].comments = (char *) malloc( 128 );
students[1].comments[51] = 'X';
return 0;
}
GDB commands
set verbose off
set pagination off
start
set logging file output/gdb_pretty_print.gdb
set logging overwrite on
set logging on
echo print students with pretty off\n
set print pretty off
print students
echo print students with pretty on\n
set print pretty on
print students
echo Printing and watching elements of arrays\n
# 10 character max width
print -elements 10 -- students[1].comments
# All character
print -elements unlimited -- students[1].comments
print students[1].comments[5]@10
next
print students[1].comments[50]@4
watch students[1].comments[50]@4
continue
set logging off
quit
# vim: ft=gdb
GDB output
print students with pretty off
$1 = {{first_name = 0x555555556008 "Fred", last_name = 0x55555555600d "Smith", dob = {day = 1, month = 1, year = 1970}, comments = 0x0}, {first_name = 0x555555556013 "Sarah", last_name = 0x555555556019 "G0T0", dob = {day = 1, month = 1, year = 2002}, comments = 0x555555556020 "Lorem ipsum enchanta constrata"}}
print students with pretty on
$2 = {{
first_name = 0x555555556008 "Fred",
last_name = 0x55555555600d "Smith",
dob = {
day = 1,
month = 1,
year = 1970
},
comments = 0x0
}, {
first_name = 0x555555556013 "Sarah",
last_name = 0x555555556019 "G0T0",
dob = {
day = 1,
month = 1,
year = 2002
},
comments = 0x555555556020 "Lorem ipsum enchanta constrata"
}}
Printing and watching elements of arrays
$3 = 0x555555556020 "Lorem ipsu"...
$4 = 0x555555556020 "Lorem ipsum enchanta constrata"
$5 = " ipsum enc"
66 students[1].comments[51] = 'X';
$6 = "\000\000\000"
Hardware watchpoint 2: students[1].comments[50]@4
Hardware watchpoint 2: students[1].comments[50]@4
Old value = "\000\000\000"
New value = "\000X\000"
main () at gdb_pretty_print.cpp:67
67 return 0;
Warning: 'set logging off', an alias for the command 'set logging enabled', is deprecated.
Use 'set logging enabled off'.