C++ if with initializer
Date: 2023-02-22Last modified: 2023-02-27
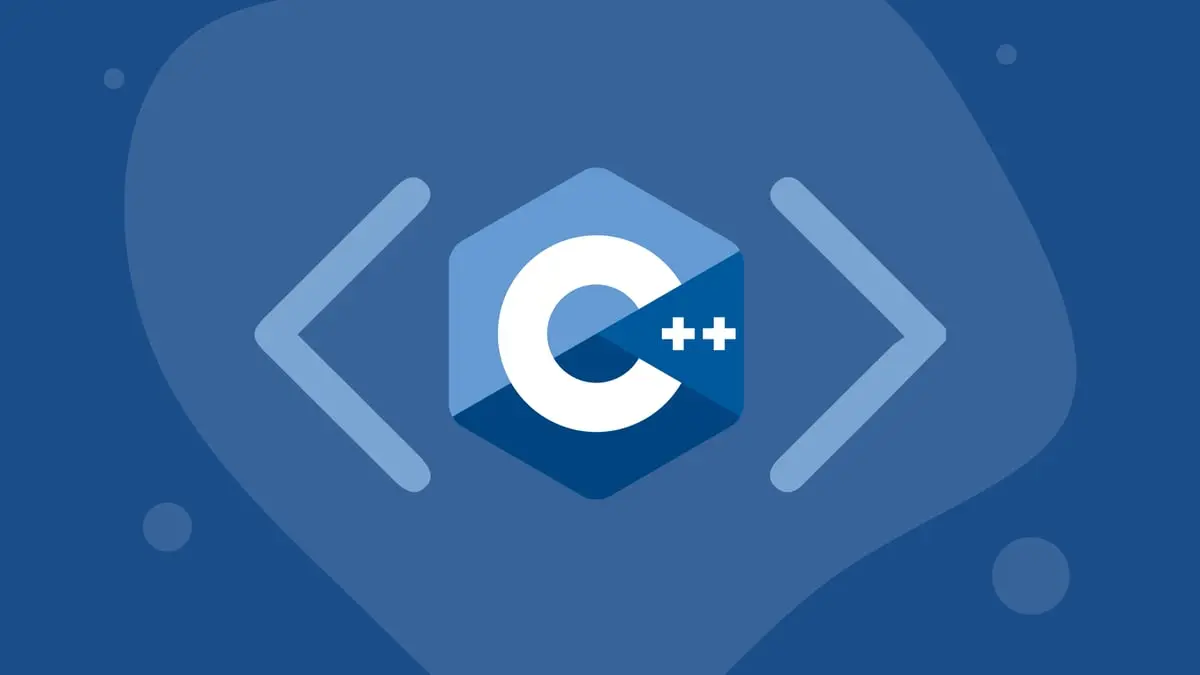
Table of contents
const int Pen{10};
const int Marker{20};
const int Eraser{30};
const int Rectangle{40};
const int Circle{50};
const int Ellipse{60};
int main([[maybe_unused]] int argc, [[maybe_unused]] char **argv) {
bool go{false};
// initialized inplace. Only one variable is allowed
if (int high_speed{33}; go) {
// high_speed is visible at if
if (high_speed > 5) {
cout << "Slow down" << endl;
} else {
cout << "All good!" << endl;
}
} else {
// high_speed is visible at else
cout << "high_speed: " << high_speed << endl;
cout << "Stop" << endl;
}
// not visible outside block
// cout << "high_speed: " << high_speed << endl;
int tool{Eraser};
// switch accept ONE initializer like if
switch (double strength{3.56}; tool) {
case Pen: {
std::cout << "Active tool is Pen. strength : " << strength << std::endl;
} break;
case Marker: {
std::cout << "Active tool is Marker. strength : " << strength
<< std::endl;
} break;
case Eraser:
case Rectangle:
case Circle: {
std::cout << "Drawing Shapes. strength : " << strength << std::endl;
} break;
case Ellipse: {
std::cout << "Active tool is Ellipse. strength : " << strength
<< std::endl;
} break;
default: {
std::cout << "No match found. strength : " << strength << std::endl;
} break;
}
return 0;
}
Possible output
high_speed: 33
Stop
Drawing Shapes. strength : 3.56