C++ lock_guard
Date: 2023-01-09Last modified: 2025-01-12
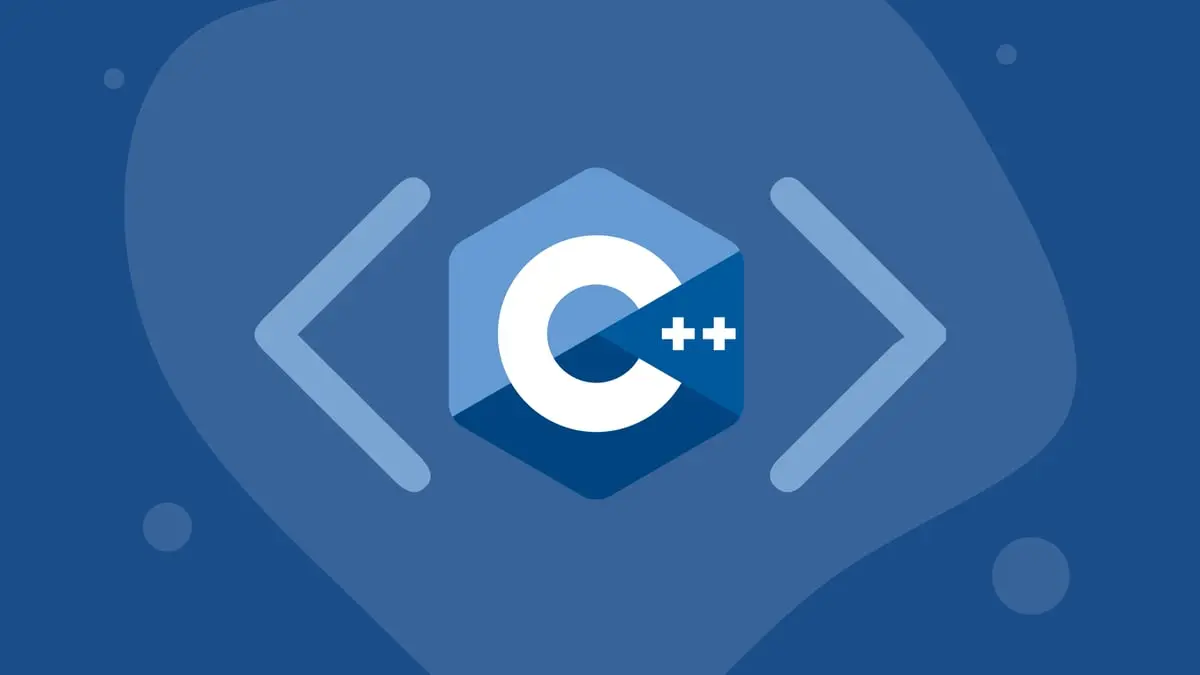
Table of contents
std::mutex mtx;
void print_even_or_throw( int x )
{
if( x % 2 == 0 ) {
fmt::print( "{} is even\n", x );
}
else {
throw( std::logic_error( fmt::format( "💥 {} is not even", x ) ) );
}
}
void print_thread_id( int id )
{
try {
// using a local lock_guard to lock mtx guarantees unlocking on destruction
std::lock_guard<std::mutex> lck( mtx );
print_even_or_throw( id );
}
catch( std::logic_error &e ) {
fmt::print( "[exception caught] {} -> {}\n", id, e.what() );
}
}
int main()
{
std::thread threads[10];
// spawn 10 threads:
for( int i = 0; i < 10; ++i ) {
threads[i] = std::thread( print_thread_id, i + 1 );
}
for( auto &th : threads ) {
th.join();
}
return 0;
}
Possible output
[exception caught] 1 -> 💥 1 is not even
10 is even
2 is even
[exception caught] 3 -> 💥 3 is not even
4 is even
[exception caught] 5 -> 💥 5 is not even
6 is even
[exception caught] 7 -> 💥 7 is not even
8 is even
[exception caught] 9 -> 💥 9 is not even