C++ lvalue_rvalue
Date: 2023-01-18Last modified: 2023-03-16
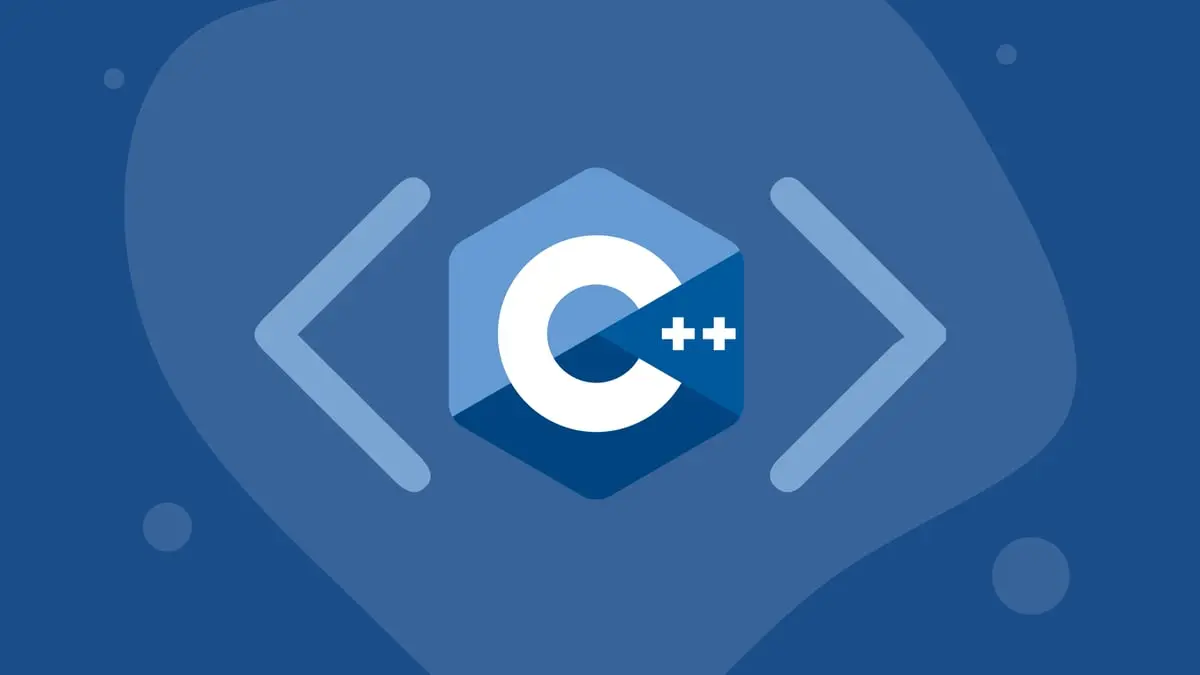
Table of contents
int GetValue() {
int value = 10;
fmt::print("GetValue() {}\n", value);
return value;
}
int& GetValue2() {
static int value = 10;
fmt::print("GetValue2() {}\n", value);
return value;
}
void SetValue(int value) { fmt::print("SetValue({})\n", value); }
This function only accept lvalues.
//-- This function only accept lvalues.
void SetValue2(int& value) { fmt::print("SetValue2({})\n", value); }
The `const` changes the behavior here to accept rvalues too.
//-- The `const` changes the behavior here to accept rvalues too.
void SetValue3(const int& value) { fmt::print("SetValue3({})\n", value); }
void PrintName(string& lvalue) {
fmt::print("Full name is: {} lvalue\n", lvalue);
}
void PrintName2(string&& rvalue) {
fmt::print("Full name is: {} rvalue\n", rvalue);
}
int a = GetValue();
fmt::print("value returned by GetValue() is {}\n", a);
// GetValue() = 10; // this operation is not possible
GetValue2() = 20; // lvalue is ok
fmt::print("Value stored into function GetValue2() is {}\n", GetValue2());
int b = 10; // b is a lvalue 10 is a rvalue
SetValue(b); // call with lvalue
SetValue(10); // call with rvalue (temporary object)
☢
Warning
You can’t take a reference from a rvalue.
SetValue2(b); // call with lvalue
// SetValue2(10); // this operation is not possible
SetValue3(10); // ok, the compiler will create a temporary variable and get a
// reference for it.
// int temp = 10; SetValue(temp);
string firstName = "Geraldo";
string lastName = "Ribeiro";
string fullName = firstName + lastName;
PrintName(fullName); // ok lvalue
// PrintName(firstName + lastName); // not ok, rvalue
// again in change the function signature to accept const string& will works
PrintName2(firstName + lastName); // ok rvalue
// PrintName2(fullName); // not ok, lvalue
## Possible output
```txt
GetValue() 10
value returned by GetValue() is 10
GetValue2() 10
GetValue2() 20
Value stored into function GetValue2() is 20
SetValue(10)
SetValue(10)
SetValue2(10)
SetValue3(10)
Full name is: GeraldoRibeiro lvalue
Full name is: GeraldoRibeiro rvalue