C++ pthread 02
Date: 2024-01-23Last modified: 2025-01-12
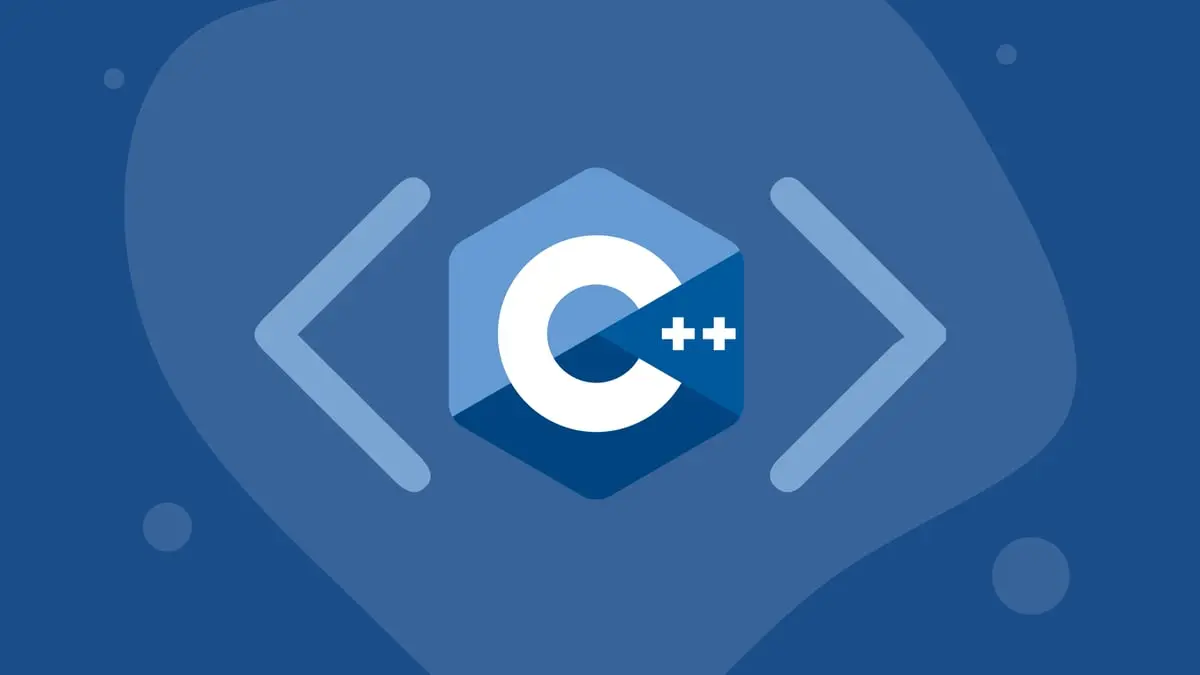
Table of contents
void *thread_callback( void *heap_argument )
{
// cancelation is define inside the thread
// it cannot be assigned to another thread
pthread_setcancelstate( PTHREAD_CANCEL_ENABLE, 0 );
pthread_setcanceltype( PTHREAD_CANCEL_ASYNCHRONOUS, 0 );
int local_argument = *(int *)heap_argument;
free( heap_argument );
while( 1 ) {
printf( "thread %d\n", local_argument );
sleep( 1 );
}
printf( "This line should never be reached\n" );
return 0;
}
void create_a_cancelable_thread( pthread_t *thread_handler, int local_argument )
{
pthread_attr_t attr;
pthread_attr_init( &attr );
pthread_attr_setdetachstate( &attr, PTHREAD_CREATE_JOINABLE );
// Allocate memory for the arguments
int *heap_argument = (int *)calloc( 1, sizeof( int ) );
*heap_argument = local_argument;
// POSIX thread creation
pthread_create( thread_handler, &attr, &thread_callback, (void *)heap_argument );
}
void cancel_thread( pthread_t thread_handler, int tid )
{
// Cancelation is async, then it does not occurs on the exact time of cancel is called
printf( "Canceling thread #%d\n", tid );
pthread_cancel( thread_handler );
}
int main( [[maybe_unused]] int argc, [[maybe_unused]] char **argv )
{
pthread_t t1;
pthread_t t2;
pthread_t t3;
create_a_cancelable_thread( &t1, 1 );
create_a_cancelable_thread( &t2, 2 );
create_a_cancelable_thread( &t3, 3 );
sleep( 2 );
cancel_thread( t1, 1 );
sleep( 2 );
cancel_thread( t2, 2 );
sleep( 2 );
cancel_thread( t3, 3 );
sleep( 1 );
return 0;
}
Possible output
thread 1
thread 3
thread 2
thread 1
thread 3
thread 2
Canceling thread #1
thread 1
thread 3
thread 2
thread 3
thread 2
thread 3
thread 2
Canceling thread #2
thread 3
Canceling thread #3