C++ tasks
Date: 2023-02-08Last modified: 2024-11-02
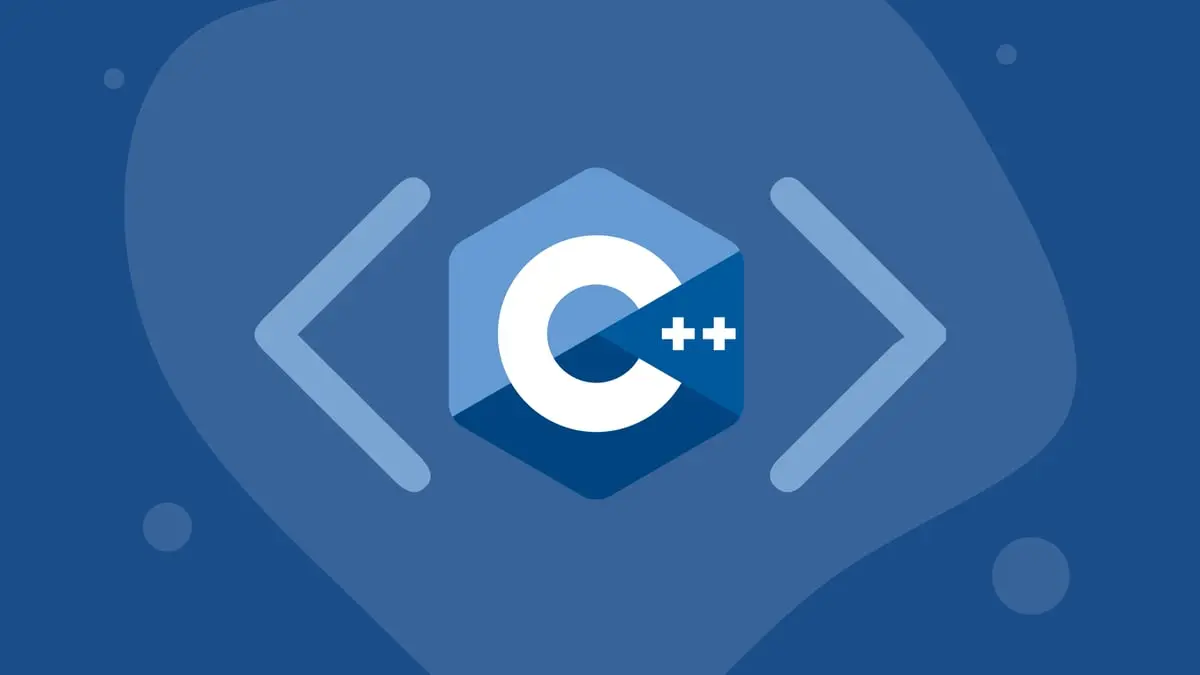
Table of contents
#include <fmt/core.h>
#include <chrono>
#include <future>
#include <iostream>
#include <thread>
#include <vector>
#include <algorithm>
int main() {
std::cout << "Main thread id: " << std::this_thread::get_id() << std::endl;
std::vector<std::future<int>> futures;
auto beg = std::chrono::high_resolution_clock::now();
for (int i = 0; i < 1000; ++i) {
auto fut = std::async([i] {
std::this_thread::sleep_for(std::chrono::seconds(1));
return i;
});
futures.push_back(std::move(fut));
}
std::for_each(futures.begin(), futures.end(),
[](std::future<int>& fut) { fut.wait(); });
auto end = std::chrono::high_resolution_clock::now();
auto duration = duration_cast<std::chrono::milliseconds>(end - beg);
std::cout << "Total duration for 1000 tasks of 1s: " << duration.count()
<< "ms" << std::endl;
}
Possible output
Main thread id: 139961737898688
Total duration for 1000 tasks of 1s: 1017ms