C++ thread 01
Date: 2024-01-22Last modified: 2025-01-12
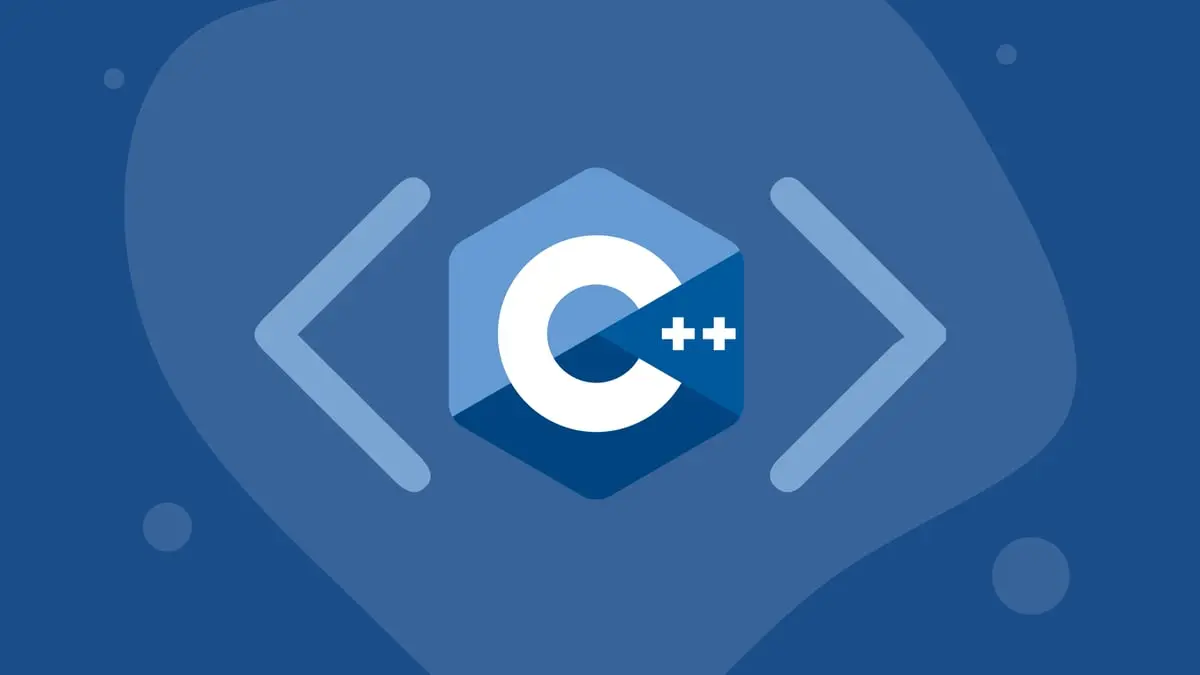
Table of contents
The std::thread
has a obvious drawback: it is simply a thread, it can
not return any value. So if we want to get something from a thread,
we can only use some global variable to exchange the result. Even we
use global variable to pass result, we still don’t know when the
result is ready, then we need one more variable as signal variable
to record the status, that’s not acceptable.
void thread_function()
{
fmt::print( "Thread start\n" );
sleep( 2 );
fmt::print( "Thread finish\n" );
// I can't return a value :(
}
int async_function()
{
fmt::print( "Async thread start\n" );
sleep( 3 );
fmt::print( "Async thread finish\n" );
return 42;
}
int main( [[maybe_unused]] int argc, [[maybe_unused]] char **argv )
{
fmt::print( "main start\n" );
std::thread t1( thread_function ); // now it's running
std::future<int> fut = std::async( std::launch::async, async_function );
// do something else ....
// Block main and wait for t1 finish
fmt::print( "joining t1\n" );
t1.join(); // before the ending, t1 should join
fmt::print( "t1 joined\n" );
int ret = fut.get(); // wait for function finishes
fmt::print( "Async ret = {}\n", ret );
fmt::print( "main finish\n" );
return 0;
}
Possible output
main start
joining t1
Thread start
Async thread start
Thread finish
t1 joined
Async thread finish
Async ret = 42
main finish