C++ thread 02
Date: 2024-02-17Last modified: 2024-02-26
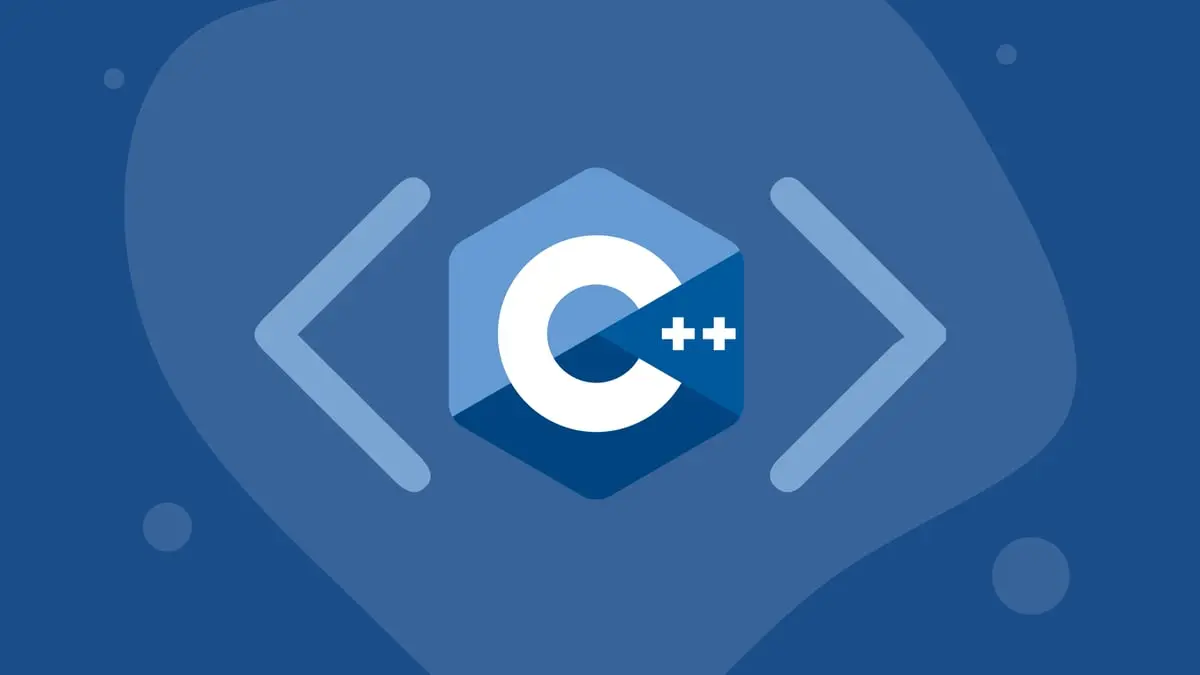
Table of contents
void func_1()
{
fmt::print( "hello from method {}\n", __FUNCTION__ );
}
void func_2()
{
fmt::print( "hello from method {}\n", __FUNCTION__ );
}
int main()
{
std::thread thread_1( func_1 );
std::thread thread_2( func_2 );
// This program is unsafe
// I intentionally forgot to join or detach here to produce an error
// thread_1.join();
thread_2.join();
if( thread_1.joinable() ) {
fmt::print( "thread_1 is joinable thread\n" );
}
if( thread_2.joinable() ) {
fmt::print( "thread_2 is joinable thread\n" );
}
else {
fmt::print( "after calling join, thread_2 is not a joinable thread\n" );
}
fmt::print( "hello from main thread\n" );
// give time for all threads execute their functions
std::this_thread::sleep_for( std::chrono::seconds( 1 ) );
std::fflush( nullptr );
// An exception std::terminate should be throwed for thread_1
return 0;
}
Possible output
hello from method func_1
hello from method func_2
thread_1 is joinable thread
after calling join, thread_2 is not a joinable thread
hello from main thread
Possible error output
terminate called without an active exception