C++ thread 03
Date: 2024-02-17Last modified: 2024-02-26
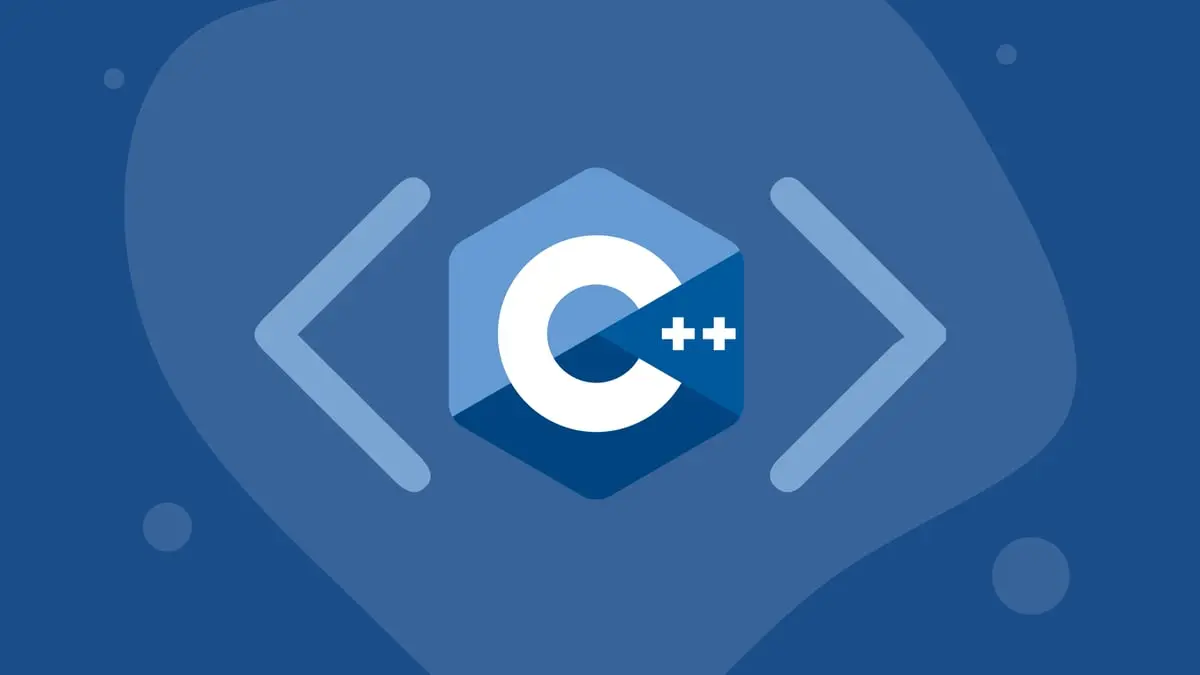
Table of contents
void func_1()
{
fmt::print( "hello from method {}\n", __FUNCTION__ );
}
int main()
{
try {
std::thread thread_1( func_1 );
// I intentionally forgot to join or detach here to produce an error
// thread_1.join();
// To protect the execution I will use the thread guard
// When this object goes out to the context the join() should be called.
// Even if a exception is thowed
thread_guard_raii thread_guard_obj( thread_1 );
// Some processing
std::this_thread::sleep_for( std::chrono::seconds( 1 ) );
// Create an error
fmt::print( "Good by cruel world\n" );
throw std::runtime_error( "Some error" );
fmt::print( "This message never should be printed\n" );
}
catch( ... ) {
fmt::print( "Exception captured\n" );
}
std::fflush( nullptr );
return 0;
}
Possible output
RAII Thread Guard Constructor
hello from method func_1
Good by cruel world
RAII Thread Guard Destructor
Thread joined
Exception captured