C++ thread_local_storage_01
Date: 2024-02-17Last modified: 2024-02-26
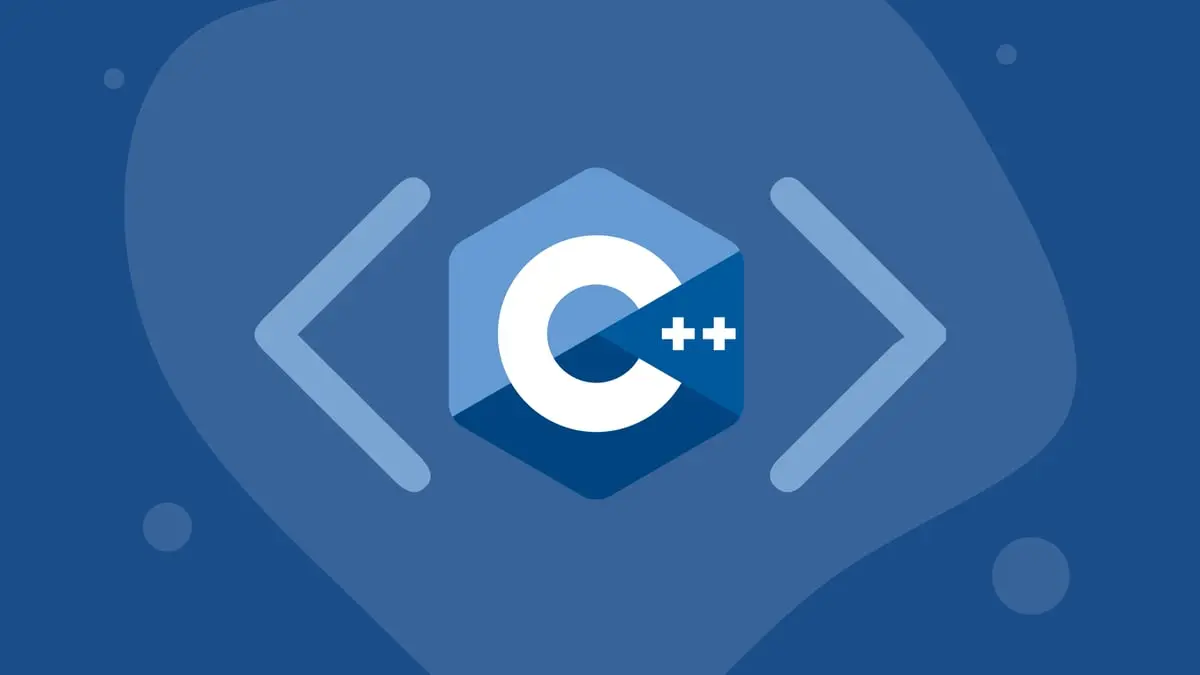
Table of contents
std::atomic<int> atomic_integer_var = 0;
thread_local std::atomic<int> atomic_integer_var_with_local_storage = 0;
void foo_1()
{
++atomic_integer_var;
fmt::print( "foo_1: {}\n", atomic_integer_var.load() );
}
void foo_2()
{
++atomic_integer_var_with_local_storage;
fmt::print( "foo_2: {}\n", atomic_integer_var_with_local_storage.load() );
}
int main( [[maybe_unused]] int argc, [[maybe_unused]] char **argv )
{
fmt::print( "All threads shares the same variable\n" );
std::thread t1( foo_1 );
std::thread t2( foo_1 );
std::thread t3( foo_1 );
t1.join();
t2.join();
t3.join();
std::thread ta( foo_2 );
std::thread tb( foo_2 );
std::thread tc( foo_2 );
ta.join();
tb.join();
tc.join();
return 0;
}
Possible output
All threads shares the same variable
foo_1: 1
foo_1: 2
foo_1: 3
foo_2: 1
foo_2: 1
foo_2: 1