C++ unique_lock 01
Date: 2024-02-17Last modified: 2024-02-26
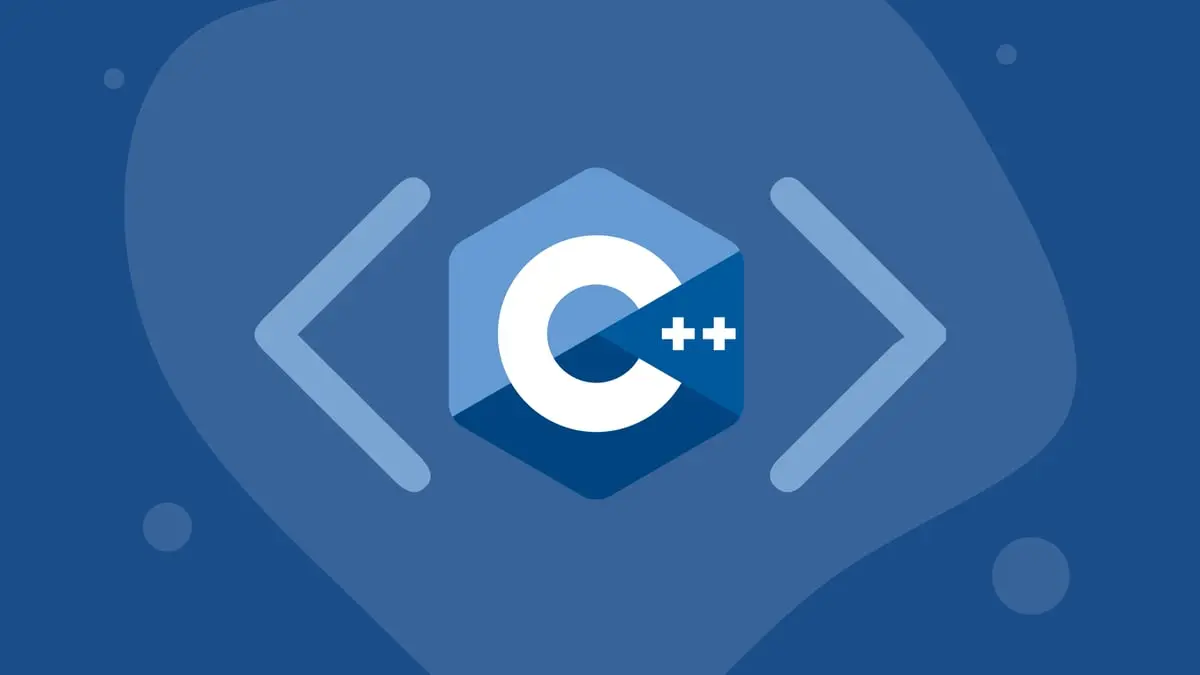
Table of contents
struct Box {
explicit Box( int num ) : num_things{ num }
{
}
int num_things;
std::mutex m;
};
void transfer( Box &from, Box &to, int num )
{
// don't actually take the locks yet
std::unique_lock lock1{ from.m, std::defer_lock };
std::unique_lock lock2{ to.m, std::defer_lock };
// lock both unique_locks without deadlock
std::lock( lock1, lock2 );
from.num_things -= num;
to.num_things += num;
// 'from.m' and 'to.m' mutexes unlocked in 'unique_lock' dtors
}
int main()
{
Box box1{ 100 };
Box box2{ 50 };
std::thread t1{ transfer, std::ref( box1 ), std::ref( box2 ), 10 };
std::thread t2{ transfer, std::ref( box2 ), std::ref( box1 ), 5 };
t1.join();
t2.join();
fmt::print( "box1: {} box2: {}\n", box1.num_things, box2.num_things );
return 0;
}
Possible output
box1: 95 box2: 55