C++ weeds
Date: 2023-02-17Last modified: 2024-11-02
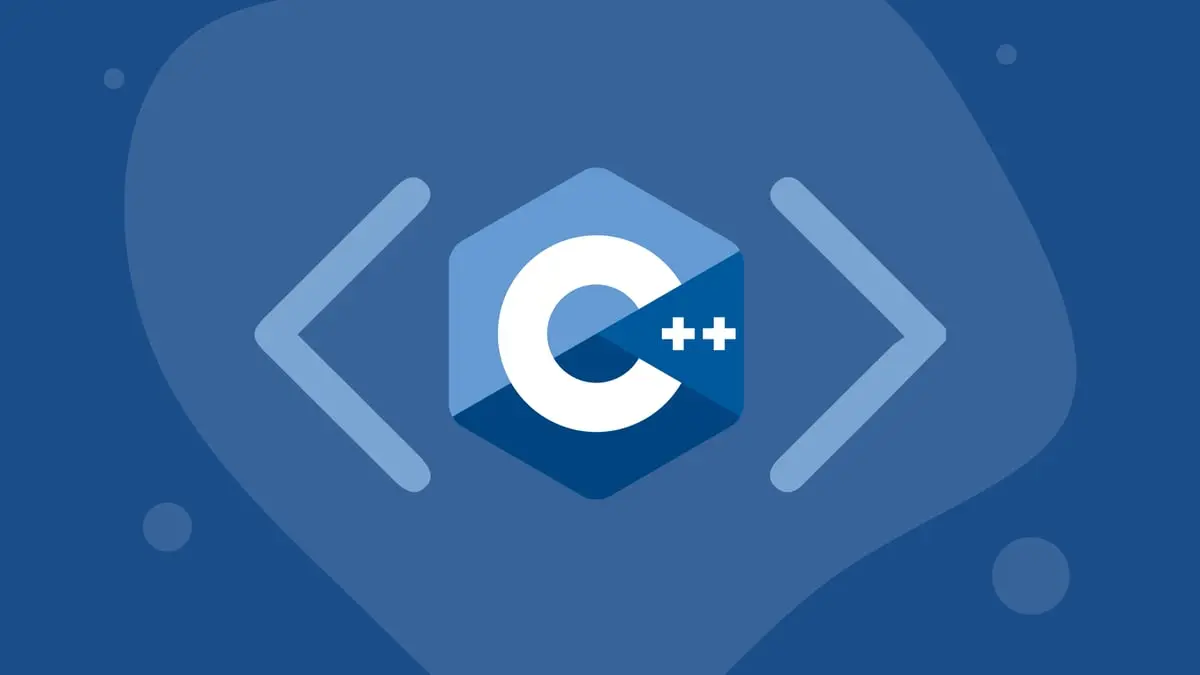
Table of contents
Introduction
☢
Warning
Don’t use in production
Operator Order of Evaluation
[intro.execution]/10
"Except where noted, evaluations of operands of individual operators and of subexpressions of individual expressions are unsequenced. The value computations of the operands of an operator are sequenced before the value computation of the result of the operator.’;[expr.comma]/1
"A pair of expressions separated by a comma is evaluated left-to-right; the left expression is a discarded-value expression. The left expression is sequenced before the right expression[expr.ass]/1
“In all cases, the assignment is sequenced after the value computation of the right and left operands, and before the value computation of the assignment expression. The right operand is sequenced before the left operand.
void ___(auto text) { std::cout << "\n--- [" << text << "]" << std::endl; }
void weeds01() {
auto print_one = [](auto t) {
std::cout << t << std::endl;
return std::type_identity<void>{};
};
___("print_one(hello) , print_one(world)");
print_one("hello"), print_one("world"); // hello world
___("print_one(hello) = print_one(world)");
print_one("hello") = print_one("world"); // world hello
auto fold_print_assign_right = [&](auto... vals) { (print_one(vals) = ...); };
auto fold_print_assign_left = [&](auto... vals) { (... = print_one(vals)); };
___("fold_print_assign_right(1,2,3,4)");
fold_print_assign_right(1, 2, 3, 4); // 4 3 2 1
___("fold_print_assign_left(1,2,3,4)");
fold_print_assign_left(1, 2, 3, 4); // 4 3 2 1
auto fold_print_comma_right = [&](auto... vals) { (print_one(vals), ...); };
auto fold_print_comma_left = [&](auto... vals) { (..., print_one(vals)); };
___("fold_print_comma_right(1,2,3,4)");
fold_print_comma_right(1, 2, 3, 4); // 4 3 2 1
___("fold_print_comma_left(1,2,3,4)");
fold_print_comma_left(1, 2, 3, 4); // 4 3 2 1
}
Possible output
--- [print_one(hello) , print_one(world)]
hello
world
--- [print_one(hello) = print_one(world)]
world
hello
--- [fold_print_assign_right(1,2,3,4)]
4
3
2
1
--- [fold_print_assign_left(1,2,3,4)]
4
3
2
1
--- [fold_print_comma_right(1,2,3,4)]
1
2
3
4
--- [fold_print_comma_left(1,2,3,4)]
1
2
3
4