C++ zeromq push
Date: 2020-11-03Last modified: 2024-06-09
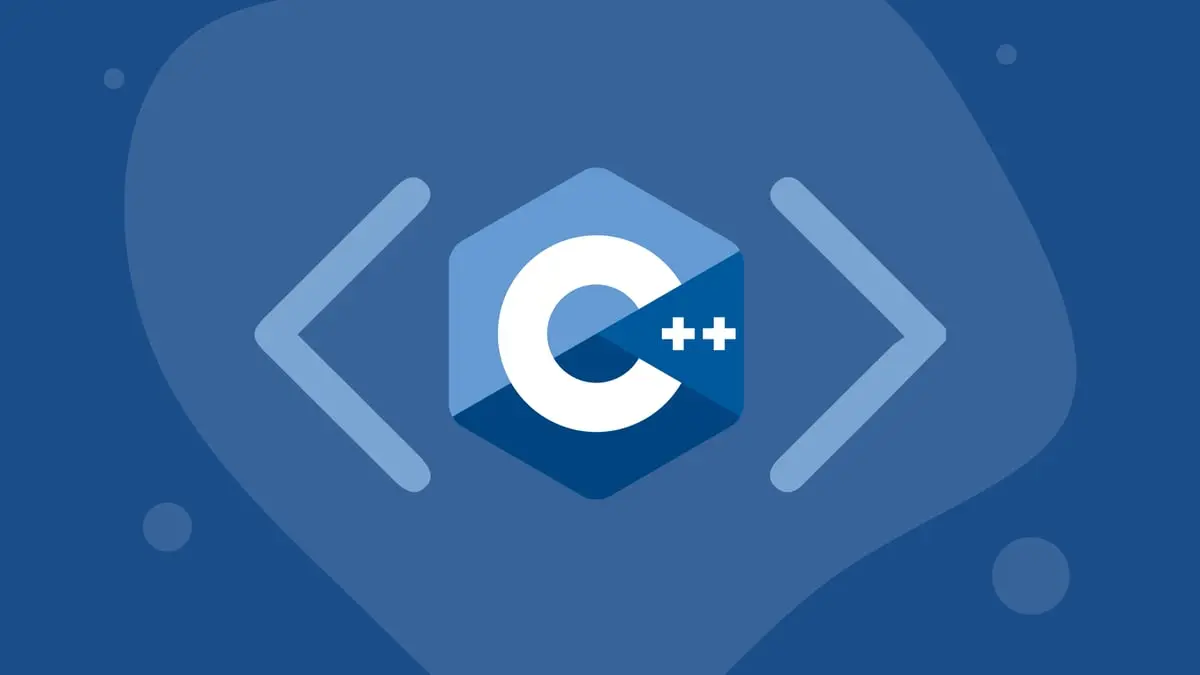
Table of contents
#include <spdlog/spdlog.h>
#include <future>
#include <iostream>
#include <string>
#include <zmq.hpp>
#include <zmq_addon.hpp>
int main()
{
zmq::context_t ctx;
zmq::socket_t pusher( ctx, zmq::socket_type::push );
//pusher.connect( "ipc://tmp/x" );
pusher.connect( "tcp://127.0.0.1:15555" );
// Give the pullers a chance to connect, so they don't lose any messages
std::this_thread::sleep_for( std::chrono::milliseconds( 20 ) );
for( auto i=0; i<10; ++i ) {
// Write three messages, each with an envelope and content
pusher.send( zmq::str_buffer( "A" ), zmq::send_flags::sndmore );
pusher.send( zmq::str_buffer( "Message in A envelope" ) );
pusher.send( zmq::str_buffer( "B" ), zmq::send_flags::sndmore );
pusher.send( zmq::str_buffer( "Message in B envelope" ) );
pusher.send( zmq::str_buffer( "C" ), zmq::send_flags::sndmore );
pusher.send( zmq::str_buffer( "Message in C envelope" ) );
std::this_thread::sleep_for( std::chrono::milliseconds( 500 ) );
}
return 0;
}
Possible output
xxx