C++ exception — 01
Date: 2023-01-09Last modified: 2023-01-13
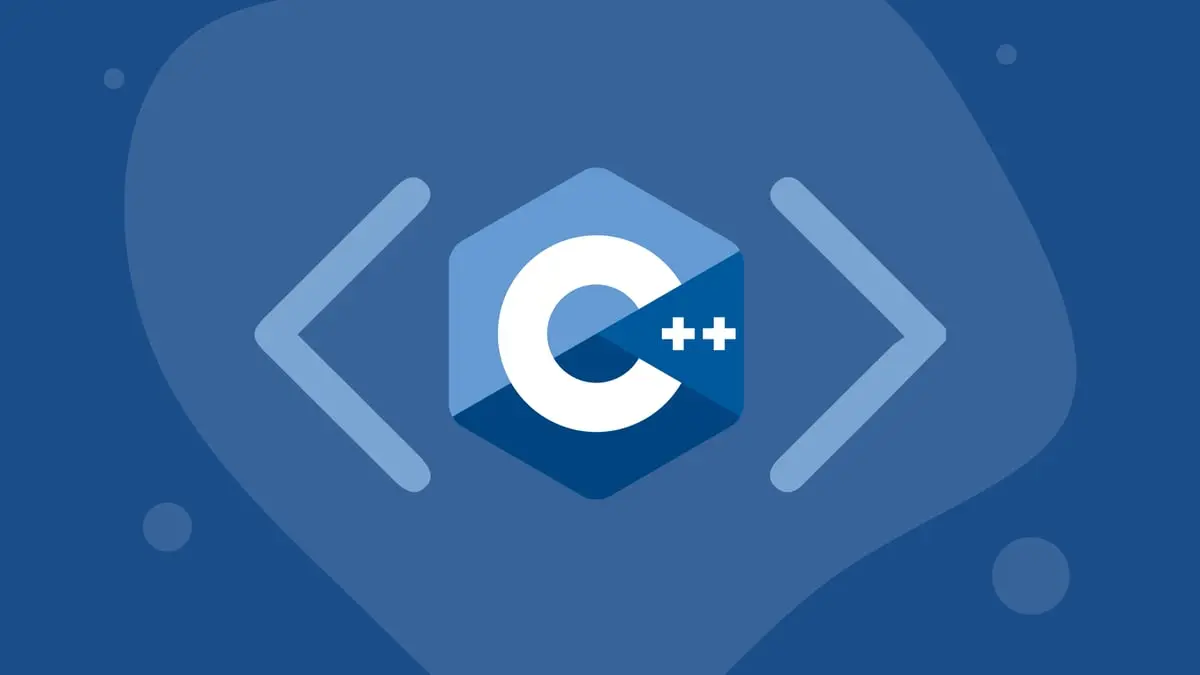
Table of contents
Strong and weak exception guarantee
When writing any C++ code, we have essentially four options regarding exceptions:
- we can completely ignore exceptions (no exception guarantee)
- we can guarantee that our code does not throw exceptions (noexcept)
- we can guarantee that if our code throws, it doesn’t violate any invariants (weak exception guarantee)
- we can guarantee that if our code throws, the state doesn’t change (strong exception guarantee)
The strong exception guarantee is effectively transactional. Either the operation succeeds, or nothing changes.
The weak exception guarantee permits partial changes (that do not violate invariants).
This topic is complex, and was divided into several parts.
#include <fmt/core.h>
#include <stdexcept>
void error_maker() { throw std::runtime_error("This is a runtime error."); }
int main() {
try {
error_maker();
} catch (std::exception& e) {
fmt::print("Failed: {}\n", e.what());
}
return 0;
}
Possible output
Failed: This is a runtime error.